Overview
This brief article provides two simple C/C++ programs that create and display a raw image test pattern (dark to light gradient). The display program makes use of the OpenCV 4 libraries.
Make Raw
The program shown below creates a single pixel (RAW) test pattern and writes it out to a binary file. Two #define's are used to specify the columns and rows. The example generates a 640x480 (SD) image.
#include <iostream> #include <fstream> #include <string> using namespace std; // COLS pixels per line, and ROWS lines per frame #define COLS 640 #define ROWS 480 int main( int argc, char** argv ) { unsigned char pixel; int row = 0; int col = 0; unsigned char buffer[ROWS*COLS]; ofstream outFile(argv[1], ios::out | ios::binary); for (int i =0; i < ROWS*COLS ; i++) { pixel = (unsigned char) (col++ >> 2); outFile << dec << pixel; if (col==COLS) col = 0; } outFile.close(); return 0; }
We build it and run it as shown below:
$ gcc -g make_raw.cpp -lstdc++ -o make_raw $ ./make_raw image.raw $ file image.raw image.raw: data $ hexdump image.raw | more 0000000 0000 0000 0101 0101 0202 0202 0303 0303 0000010 0404 0404 0505 0505 0606 0606 0707 0707 0000020 0808 0808 0909 0909 0a0a 0a0a 0b0b 0b0b 0000030 0c0c 0c0c 0d0d 0d0d 0e0e 0e0e 0f0f 0f0f 0000040 1010 1010 1111 1111 1212 1212 1313 1313 ...
Show Raw
For displaying the RAW image, we make use of OpenCV. The show_raw program is shown below:
#include <opencv2/opencv.hpp> #include <iostream> #include <fstream> #include <string> #include <stdio.h> using namespace std; #define ROWS 480 #define COLS 640 void help(char** argv ) { std::cout << "\n" << "loads and displays a raw 8-bit pixel image\n" << argv[0] <<" <path/filename>\n" << std::endl; } int main( int argc, char** argv ) { unsigned char pixel; int row = 0; int col = 0; if (argc != 2) { help(argv); return 0; } // row, col unsigned char buffer[ROWS*COLS]; FILE *fptr; fptr = fopen(argv[1],"rb"); fread(&buffer,1,ROWS*COLS,fptr); cv::Mat img(ROWS,COLS,CV_8U,&buffer); if( img.empty() ) return -1; cv::namedWindow( "img", cv::WINDOW_AUTOSIZE ); cv::imshow( "img", img ); cv::waitKey( 0 ); while(1) { // wait for user to Ctrl C to exit } return 0; }
We build show_raw and run it as shown below:
$ gcc -g show_raw.cpp -I/opt/opencv/include/opencv4 -L/opt/opencv/lib -lstdc++ -lopencv_imgcodecs \ -lopencv_highgui -lopencv_core -lopencv_imgproc -lopencv_videoio -lopencv_video -o show_raw $ ./show_raw image.raw
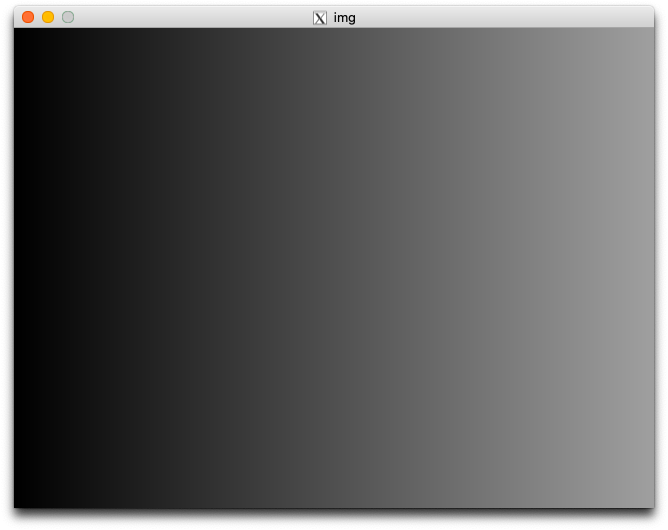